Smart Contracts Explained: An Introduction to Solidity
This blog post uncovers the concept of smart contracts with a deepdive into Solidity

In the ever-evolving world of technology, smart contracts are steadily carving a niche for themselves. This blog post uncovers the concept of smart contracts and dives deep into Solidity - the leading programming language used for writing these digital contracts. Using smart contracts, transactions and agreements can be executed automatically without the need for a middleman, revolutionizing sectors like finance, legal, healthcare, and more. However, understanding and effectively utilizing these contracts require knowledge of their structure, syntax, and data handling, along with the intricacies of gas fees on the Ethereum network. This article serves as a comprehensive guide to Solidity, from its syntax basics to coding your first smart contract and even exploring real-world use-cases of such contracts.
Understanding Smart Contracts
Let's kick off this journey by unraveling the mystery of smart contracts. In simple terms, a smart contract is a piece of computer code that runs on a blockchain platform. It's designed to automatically execute parts or the entirety of an agreement. Think of it like a virtual vending machine, where certain actions trigger specific outcomes.
To illustrate, let's say Alice wants to sell her car to Bob. In a traditional contract, they would need to get lawyers involved, sign papers and deal with the bank for payment. With a smart contract, the ownership of the car and the payment could be transferred automatically once certain conditions are met, with no need for a middleman. Cool, right?
Now, you might be wondering how smart contracts know when to execute these tasks. Well, they rely on input parameters and execution steps that are specific and objective. So, if a particular event occurs (like Bob paying the agreed price), the smart contract will execute the next step (transferring the ownership of the car to Bob).
Currently, smart contracts are primarily used for straightforward transactions, such as transferring funds between parties. However, as the adoption of blockchain spreads, we'll witness the emergence of more complex smart contracts capable of handling sophisticated transactions.
While smart contracts are a fantastic tool, it's important to note that they're not without limitations. For instance, they require a trusted, technical expert to code the agreement accurately, and non-technical parties might struggle to understand them. Despite these challenges, smart contracts offer immense potential to revolutionize various sectors by ensuring efficiency and reducing costs.
In the following sections, we'll delve deeper into Solidity, the leading programming language for writing smart contracts, and uncover how it powers these digital agreements. Buckle up, it's going to be an exciting ride!
Introduction to Solidity
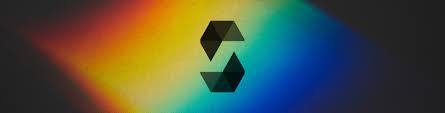
Say hello to Solidity, the superstar programming language of the blockchain universe! Born in 2015, Solidity was brought to life by Ethereum, the second-largest cryptocurrency market by capitalization. If you think of Ethereum as a superhero, then Solidity is its trusty sidekick, powering the execution of smart contracts on the Ethereum blockchain.
So, what makes Solidity so special? For starters, it's a high-level programming language. That might sound intimidating, but it simply means that it's more user-friendly and closer to natural language than low-level programming languages. This makes it simpler for us humans to read and write.
Solidity is also statically typed and object-oriented. In layman's terms, this means you have to declare your variable types upfront, and you can create objects with properties and functions - just like in real life!
Here's another cool thing: Solidity is influenced by Python, C++, and JavaScript. So, if you've dabbled in those languages before, you'll feel right at home. And even if you haven't, don't fret. Solidity's syntax is straightforward, and you'll be writing lines of code in no time.
Remember those smart contracts we talked about earlier? Well, Solidity is their mother tongue. With Solidity, you can create contracts for voting systems, crowdfunding platforms, multi-signature wallets, and more. Imagine being able to automate complex transactions without the need for intermediaries - that's what Solidity enables!
So why is Solidity important? Well, it's the primary language for blockchain platforms, running on the Ethereum Virtual Machine (EVM). Think of the EVM as a global supercomputer that executes smart contracts. Solidity is the language used to give instructions to this supercomputer.
In a nutshell, Solidity is revolutionizing the tech industry by enabling the creation and execution of smart contracts. It's a potent tool in our digital arsenal, and understanding it is a step towards a more decentralized and equitable world. So, let's dive in deeper and unlock its potential together!
Solidity Syntax Basics
Alright, folks, it's time to get our hands dirty and jump right into the nitty-gritty of Solidity syntax. Don't worry, I promise it's not as scary as it sounds. In fact, it's just like learning a new language. And don't we all love adding a new language to our repertoire?
So, what's the first thing we need to know about Solidity syntax? Well, a Solidity program is made up of contracts. A contract is similar to a class in other programming languages, and it’s where all the magic happens. Inside a contract, we can define variables and functions that interact with the Ethereum blockchain.
Here's a little taste of what a contract looks like:
Okay, next up are some basic data types. These are the building blocks for all the exciting stuff we will be doing. We have uint for unsigned integers, bool for boolean values, and string for text. There's also address which is a special type for storing Ethereum addresses. You'll use these types to define your variables, like so:
Now, let's talk about functions. Functions are the heart and soul of our contract and allow us to interact with the Ethereum blockchain. They can be viewed as globally accessible API endpoints. Each function has a name, a list of parameters, and a body. The body is where the action takes place and where you write your logic. Here's an example:
In this case, our function greet doesn't take any parameters and returns a string. The public keyword means that the function can be called from anywhere, and pure means it doesn't change the state of the contract.
And there you have it, a quick and dirty guide to Solidity syntax. We've barely scratched the surface, but hopefully, this gives you a sense of what you're dealing with. Stay tuned for more in-depth dives into data handling and gas optimization in the upcoming sections. Happy coding!
Understanding Data in Solidity
Just as we have different categories for sorting things in our daily lives, Solidity also uses various data types to keep things organized. Understanding these types is crucial because they form the backbone of any Solidity code you'll write. Let's explore them!
First off, we've got value types. These are basic data types like unsigned integers (uint), booleans (bool), and addresses (address). When we pass these types around, they are copied. Just like sharing a picture with a friend, your friend gets a copy, and the original stays with you.
Now, there are also complex types, which are a bit more nuanced. These include arrays, structs, and mappings. When we pass these types around, we're actually sharing a reference to the original. Think of it like sharing a book. If your friend makes notes in the book, those notes also appear in your copy.
Solidity is a statically typed language, meaning you need to declare the data type of a variable upfront. It's like telling your friends what kind of potluck dish you'll bring before the party. This allows for checks at compile time, reducing runtime errors and making debugging easier.
Solidity also has a unique aspect to its data system: storage and memory. Storage is where all contract state variables reside, like the permanent storage on your computer. Memory, on the other hand, is temporary and erased between external function calls, just like your computer's RAM. Understanding the difference can help optimize gas costs and improve contract efficiency.
Finally, a word on data modeling. When designing your smart contracts, it's crucial to understand that data stored in Ethereum has a fixed schema and layout. This immutability calls for careful planning and thoughtful design to ensure that your contracts can evolve and scale over time.
In summary, Solidity's data system is a vital part of the language, with its own quirks and features. Mastering it is key to writing effective, efficient smart contracts. So, keep these concepts in mind as we continue our journey!
Decoding Gas Fees
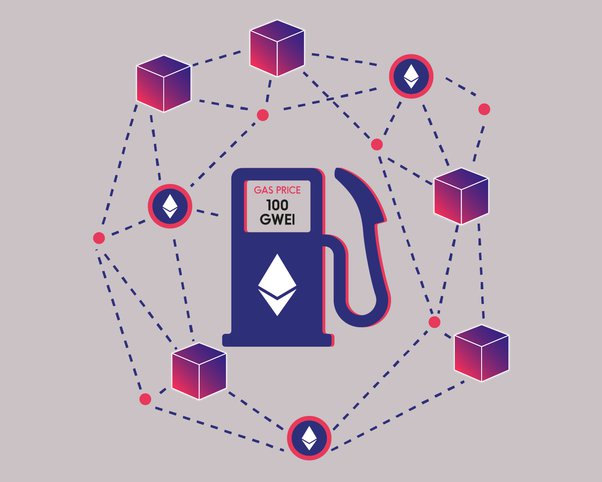
Hang on tight, because we're going to dive into one of the most critical aspects of the Ethereum network: gas fees. It may sound a bit intimidating, but trust me, it's super important and not as complex as you might think.
So what's this gas thing all about? Well, when you execute a function in Solidity, it uses computational power and resources from the Ethereum network. Just like driving a car consumes fuel, running functions on the Ethereum blockchain consumes gas. This gas isn't free, and that's where gas fees come in.
Gas fees are like a toll fee for using the Ethereum superhighway. They're measured in tiny fractions of Ethereum's native cryptocurrency, Ether, called gwei. The exact fee or price for the gas depends on various factors, like the supply and demand of the network at the time of the transaction. Think of it like surge pricing in ride-hailing apps, where prices go up when demand is high.
You might be wondering why we need these fees. Well, they serve a couple of key purposes. First, they compensate the folks who maintain and secure the Ethereum network (referred to as validators or miners). Second, they act as a deterrent against spammy or pointless transactions that could bog down the network.
Understanding gas fees is crucial because they can affect how you design and optimize your smart contracts. If a function consumes too much gas, it could become prohibitively expensive to run, especially when the network is busy. So, when writing your smart contracts, you need to be mindful of the gas costs and find ways to optimize them.
Now, I know this sounds like a lot to take in, but don't worry, we'll explore these concepts in more detail in the following sections. By the end of this, you'll be a gas fee guru, ready to optimize your smart contracts like a pro! Let's continue this exciting journey together.
Coding Your First Smart Contract
Alright, friends! Now that we've grasped the basics of Solidity, it's time to roll up our sleeves and code our first smart contract. Don't worry, I'll be here guiding you every step of the way.
To begin, we'll be using an online integrated development environment called Remix-IDE. It's an excellent platform for developing, testing, and deploying smart contracts using Solidity.
First, head over to the Remix-IDE website and create a new file with the .sol extension. This is where we'll write our smart contract. For our first contract, we'll create a simple Increment-Decrement contract. Here's how it looks:
In this contract, we define a public unsigned integer count, and two functions, increment and decrement, which increase and decrease the value of count respectively.
Once you're done writing the code, it’s time to compile it. Go to the Solidity Compiler tab in Remix-IDE, select the version of Solidity you're using, and hit the "Compile" button. This will convert your code into bytecode that can be executed on the Ethereum network. After compiling, you can view the bytecode and the Application Binary Interface (ABI) in the Compiled Contracts section.
The final step is deploying the contract. For this, we'll use the Ethereum network. Head over to the Deploy & Run Transactions tab, and hit the Deploy button. Voila! Your first smart contract is now live on the Ethereum network!
Remember, practice makes perfect. So, don't hesitate to experiment with different contracts and functionalities. As you get more comfortable, you'll realize the power you hold in your hands. You're not just coding, you're creating decentralized solutions for a more equitable world. Keep going, you're doing great!
Smart Contract Use-Cases
Alright, folks, it's time to take a look at some real-world examples of smart contracts. We've learned what they are, how they're coded, and even written our own. Now, let's explore how they're used in various sectors, solving complex issues and revolutionizing traditional systems.
Let's start with the finance sector, a pioneer in adopting blockchain tech. Smart contracts are serving up a new, decentralized financial system, called DeFi (Decentralized Finance). They're helping to automate trading, investing, lending, and borrowing, eliminating the need for intermediaries and reducing fees. This is a game-changer for those who've faced barriers to traditional financial services.
Next up is the legal industry, where smart contracts hold promising potential. They're being used to create legally binding agreements, similar to the ones that govern most of today's business dealings. Just as e-signatures revolutionized legal agreements, smart contracts are set to take it a step further, potentially reducing the costs of lawyers and other intermediaries.
In the healthcare industry, smart contracts are proving to be incredibly useful. They help share vital data across institutions, a key aspect of effective clinical trials. The blockchain's decentralized nature ensures data accuracy and reliability, a game-changer for researchers and healthcare providers.
Finally, smart contracts are finding their way into emerging technologies like AI and machine learning. By combining the data-intensive processing of AI with the decentralized security and immutability of blockchain, we could soon see AI-powered smart contracts. This could pave the way for complex computational tasks and more advanced applications of this technology.
These real-world examples are just a glimpse into the potential of smart contracts. As adoption spreads, we're likely to see even more innovative and complex use-cases across different industries. The future is bright for smart contracts, and we're only just scratching the surface!
Boosting Blockchain with ApeConsulting
Alright, folks! We've taken a deep dive into the world of smart contracts, explored Solidity, and even created our own contract. Now, let's talk about how you can take this knowledge to the next level with a little help from our friends at ApeConsulting.
ApeConsulting is a leading pioneer in the blockchain ecosystem, offering a wide array of services from IT consulting to custom software development. They have a team of experts who live and breathe blockchain, and they're here to guide you in navigating this exciting world.
If you're looking to implement smart contracts, ApeConsulting has got you covered. Whether you're in finance, legal, healthcare, or any other industry, they can create bespoke smart contract solutions tailored to your needs. Plus, they'll help you understand and optimize gas fees, making your contracts more efficient and cost-effective.
But that's not all! ApeConsulting goes beyond just smart contracts. They offer comprehensive development services for all parts of the blockchain applications for business. Whether it's front-end or back-end, they use cutting-edge technologies to build robust and scalable products.
To top it all off, they also provide expert advice on decentralized technology and Web3 products. So, if you're looking to explore the potential of Web 3.0, they're your go-to guides. With their deep understanding and extensive experience, they can help you unlock the full potential of these emerging technologies.
In a nutshell, ApeConsulting is your one-stop-shop for all things blockchain. They offer the expertise, the tools, and the passion to help you make the most of this revolutionary technology. So, why wait? Explore their services, get in touch, and start your blockchain journey with ApeConsulting today! After all, the future belongs to those who dare to embrace the new, and with ApeConsulting, you're in good hands.
This blog post provided a detailed exploration of smart contracts and the Solidity language. It took you through the basics of Solidity syntax, understanding data types, decoding gas fees in the Ethereum network, and culminated in coding your first smart contract. We also explored how smart contracts are making their mark in sectors like finance, legal, and healthcare, among others. The world of smart contracts holds vast potential and understanding them is a step towards a more efficient and decentralized future. So, keep exploring, keep learning, and step confidently into the exciting world of blockchain technology.
Frequently Asked Questions
What is Solidity and why is it important?
Solidity is a high-level programming language used for writing smart contracts on blockchain platforms, primarily Ethereum. It is crucial as it enables the creation and execution of smart contracts, thereby automating complex transactions and reducing the need for intermediaries.
What are gas fees in the Ethereum network?
Gas fees are like toll fees for using the Ethereum network. When a function in Solidity is executed, it uses computational power and resources, consuming 'gas'. These fees compensate the network maintainers and deter spammy or pointless transactions.
How are smart contracts being used in various industries?
Smart contracts are being increasingly used in sectors like finance (for automating trading, investing, etc.), legal (for creating binding agreements), healthcare (for sharing vital data), and more. They help in ensuring efficiency, reducing costs, and eliminating intermediaries.